I've been quiet the last couple of weeks as I prepared to upgrade our Maximo environment to 7.5. Last week I finally made the move with a marathon session on Wednesday with the upgrade and then did triage Thursday. With a new version comes new features. The one I'm looking forward to the most is Automation Scripting.
IBM's definition of Automation Scripting is:
An automation script consists of a launch point, variables with corresponding binding values, and the source code. You use wizards to create the components of an automation script. You create scripts and launch points or you create a launch point and associate the launch point with an existing script.1
In typical geek fashion, the definition lacks the emotional punch of what's actually being described. What Automation Scripting does is replace the burden of creating a custom java class file Maximo that does a specific function or an Escalation that had to run so frequently it would try catch records almost instantaneously. But getting started with Automation Scripting can be a little daunting. Here's a quick primer to get you started.
Disclaimer:
Most (ok... all) of this came from a great session I had with Brian Baird. Brian's very active on the developerWorks forums and the Maximo Manufacturer's User Group. Hit him up on Twitter... he'll love the attention.
Background
The first time I tried to use Automation Scripting was to set the Scheduled Start/Finish dates on a work order based on the Assignment dates. This was supposed to work out of the box. So when I tried it the firsts time, guess what happened? Pfffttt... nothing.
This should have been a slam dunk to get working because it came from IBM, right? Apparently I wasn't as smart as I thought I was. Since this was my first Automation Script I was still getting used to the setup and debugging. I reached out to the Maximo user community and Brian stepped up to answer a few of my questions. His suggested development path is what got the script working for me.
Start with the basics
An Automation Script has three basic points:
- The launch point - context of where/how the script is initiated
- The variables - input/output variables to the script
- The script - executes the functions on the input and output variables
If these three components don't work together, then your script won't work no matter how well it's written.
The next step to getting your scripting done right is to start off with the right tools. In this case Notepad ++. This is one of my must have utilities on any Maximo server I run. Notepad++ also has the ability to turn on syntax highlighting. In this case we want to use the Python syntax.
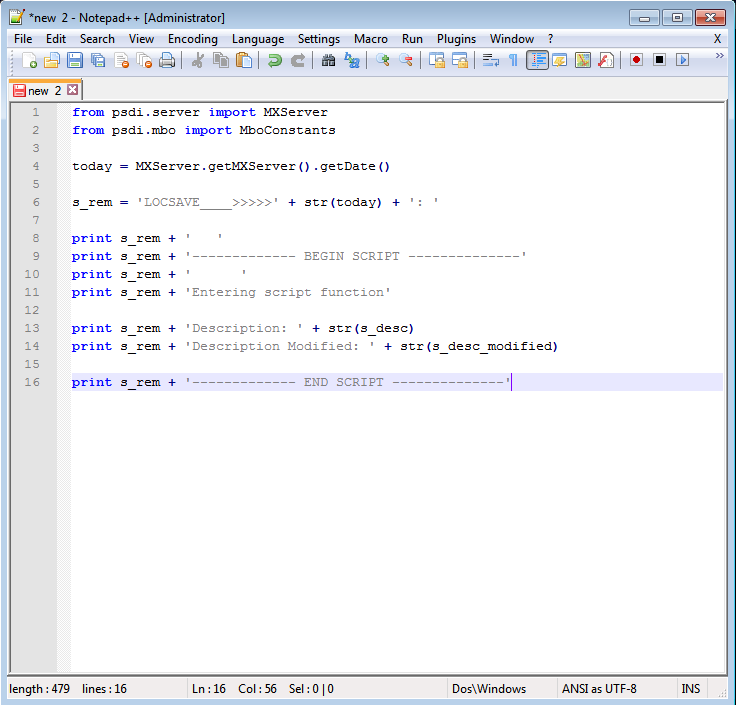
I'll get into the script itself in the next section.
Laying the ground work
When I talked to Brian about the problems I was having, he asked if I was able to confirm if the Automation Script was even firing off. Nope, I couldn't. He walked me through the basics to ensure your Automation Script is firing off. This part was exactly what I skipped when I tried to apply the 'Set Scheduled Start/Finish Date' script.
- Create a separate log appender to capture just Automation Script issues.
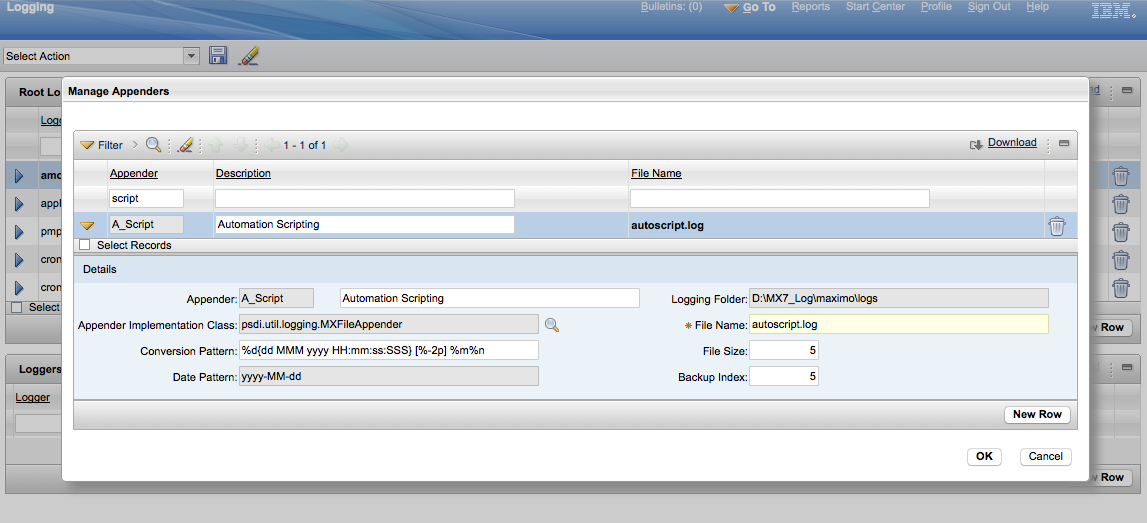
- Set the
autoscript
logger to show log output to the appender created in the previous step.
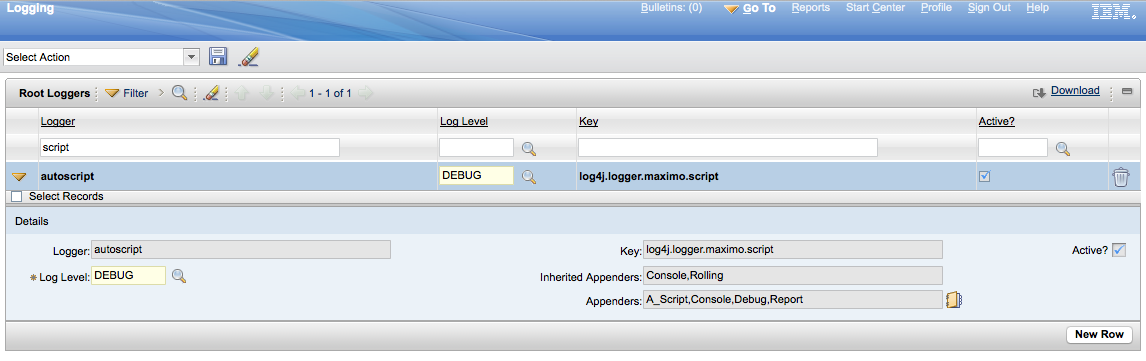
- Go to System Configuration > Platform Configuration > Automation Scripts to create a new Automation Script.
- From the Select Action menu, click Creat > Script with Object Launch Point.

- Create a name for the launch point -
LOC_SAVE
- against theLOCATIONS
object. Make sure to check theAdd?
andUpdate?
events. Then click Next.
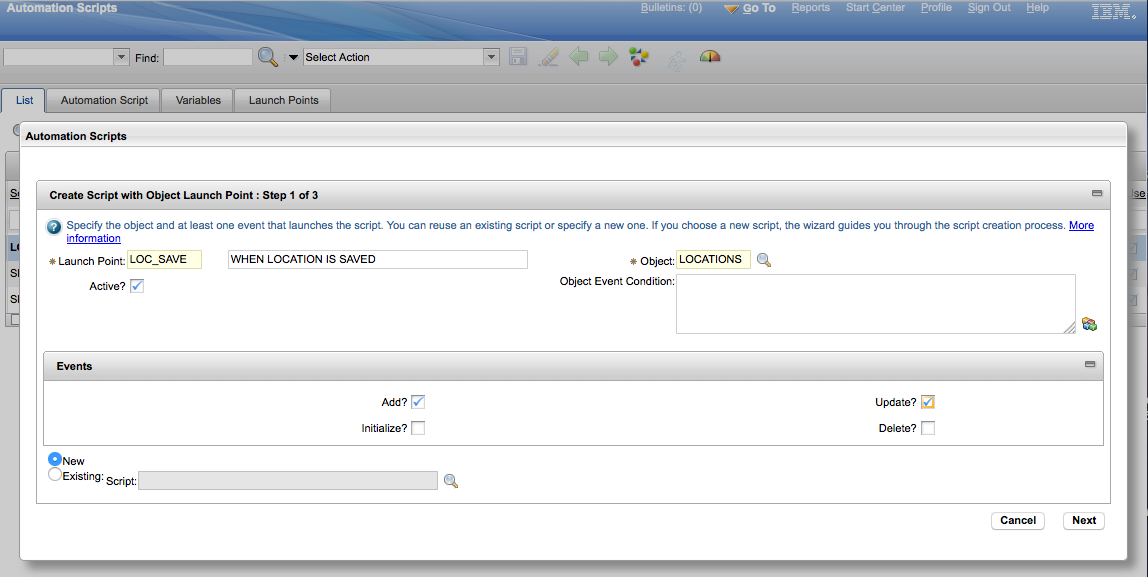
- On the 'Create Script' tab, even though you don't have your script yet, give it a name to load it in later. In this case I'll be using the
jython
language. - Now we need to enter the variables that will be used in script to interact with Maximo. It is critical that your variables match character to character here as they will in your script. In the example the variable is labeled
description_t
. Click Next.
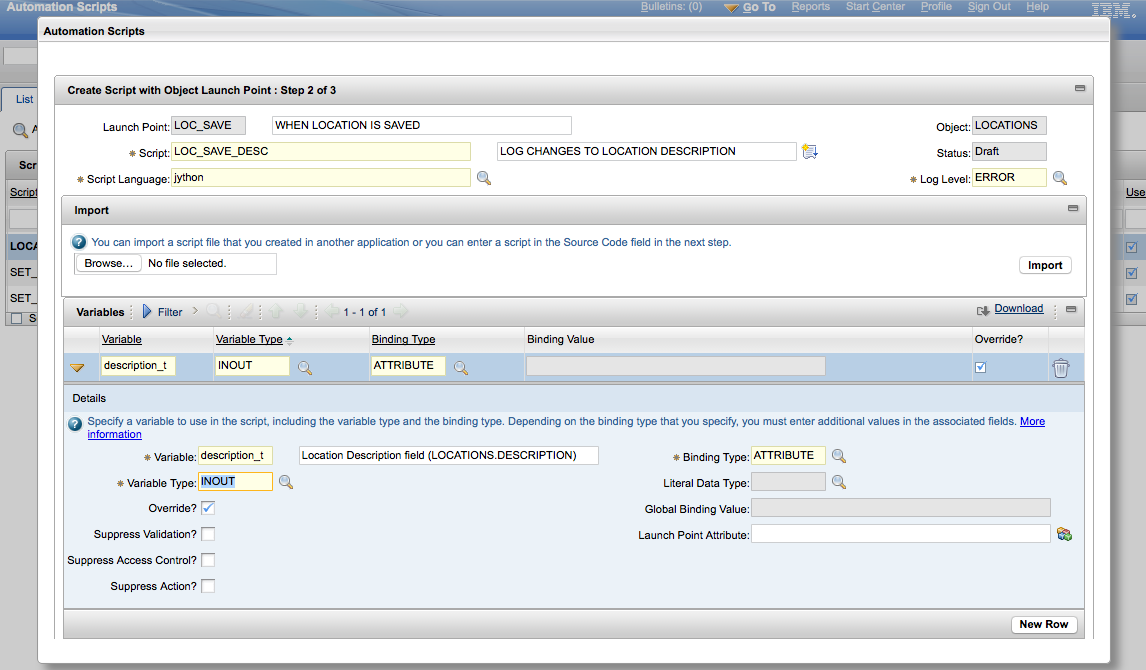
-
The 'Create Script' tab is where we can add the actual script file. In the beginning I'm going to add just the basics to prove that the script is getting picked up by Maximo and getting triggered - this was the heart of Brian's advice and it makes total sense. Brian suggested that you don't add the functionality to a script in the beginning, but add a general framework first. If the general framework works, then you can build the bells and whistles and know what bell or whistle broke your scrip.
The following script is general framework to prove your Automation Script is getting triggered in Maximo and write something out to the log file.
from psdi.server import MXServer from psdi.mbo import MboConstants today = MXServer.getMXServer().getDate() s_rem = 'LOC_SAVE____>>>>>' + str(today) + ': ' print s_rem + ' ' print s_rem + '------------- BEGIN SCRIPT --------------' print s_rem + ' ' print s_rem + '------------- END SCRIPT --------------' print ' '
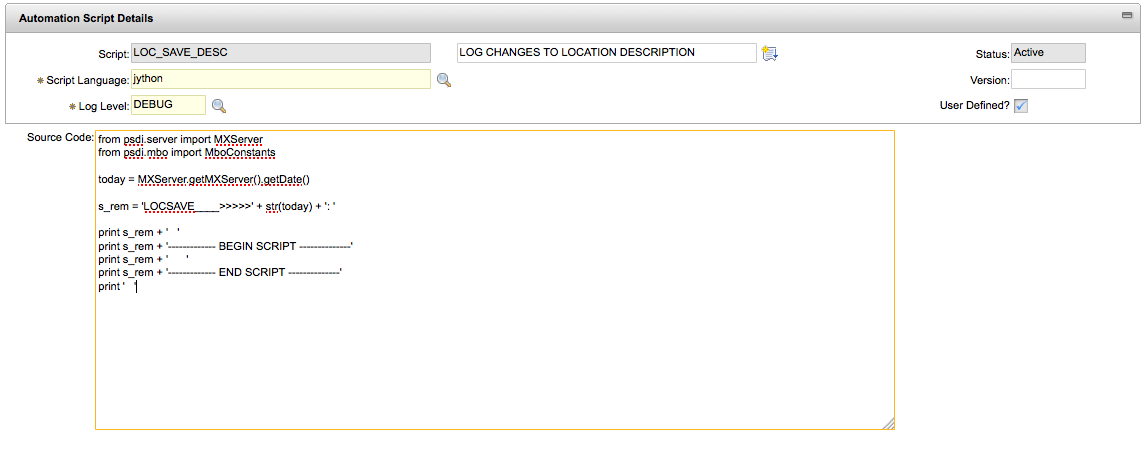
- Click the 'Create' button in the lower right to create the Automation Script.
- Verify the 'Log Level' is set to
DEBUG
.
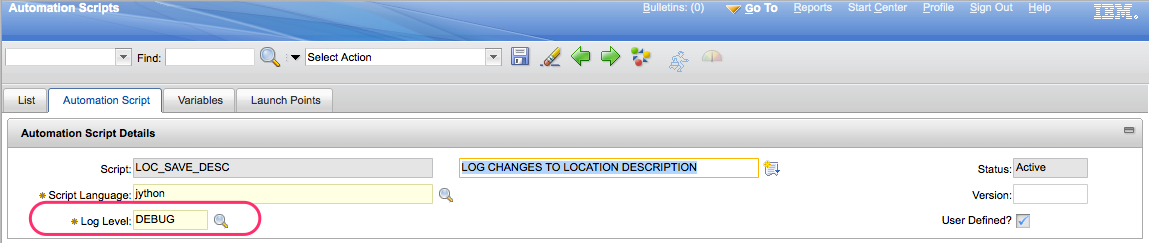
- Change the status of the script from 'Draft' to 'Active'.
- At this point we can go into the Locations app and update a description to see if the Automation script fires.
- Go to the Locations app.
- Select a Location record.
- Open the Main Tab and edit the record's Description field.
- Save the record.
- Look at the log file and you will see the following:
29 May 2015 11:21:10:631 [DEBUG] LOCSAVE____>>>>>Fri May 29 11:21:10 CDT 2015: LOCSAVE____>>>>>Fri May 29 11:21:10 CDT 2015: ------------- BEGIN SCRIPT -------------- LOCSAVE____>>>>>Fri May 29 11:21:10 CDT 2015: LOCSAVE____>>>>>Fri May 29 11:21:10 CDT 2015: ------------- END SCRIPT --------------
Extending the script
Now that I can see the script is getting triggered as expected, I can expand the script to start doing something. In this example I'll extend the script to log the new description in the log file. Going back to the original setup we included a the variable description_t
in the setup. This is variable we'll add to the script to log change out to the log file.
Open the Automation Script and update the script with two new lines:
from psdi.server import MXServer
from psdi.mbo import MboConstants
today = MXServer.getMXServer().getDate()
s_rem = 'LOC_SAVE____>>>>>' + str(today) + ': '
print s_rem + ' '
print s_rem + '------------- BEGIN SCRIPT --------------'
print s_rem + ' '
print s_rem + 'Description: ' + str(s_desc)
print s_rem + 'Description Modified: ' + str(s_desc_modified)
print s_rem + ' '
print s_rem + '------------- END SCRIPT --------------'
The two lines do the following:
'Description: ' + str(s_desc)
: outputs the new Description value to the log file.'Description Modified: ' + str(s_desc_modified)
: passes True/False value if the variable was modified.
Editing a Location record will now show the following in the log file:
29 May 2015 11:59:18:269 [DEBUG] LOCSAVE____>>>>>Fri May 29 11:59:18 CDT 2015:
LOCSAVE____>>>>>Fri May 29 11:59:18 CDT 2015: ------------- BEGIN SCRIPT --------------
LOCSAVE____>>>>>Fri May 29 11:59:18 CDT 2015:
LOCSAVE____>>>>>Fri May 29 11:59:18 CDT 2015: Description: THANKS FOR THE HELP BRIAN!
LOCSAVE____>>>>>Fri May 29 11:59:18 CDT 2015: Description Modified: True
LOCSAVE____>>>>>Fri May 29 11:59:18 CDT 2015:
LOCSAVE____>>>>>Fri May 29 11:59:18 CDT 2015: ------------- END SCRIPT --------------
Now you can add additional functionality to the script and know the script is getting triggered by Maximo.
-
http://www-01.ibm.com/support/knowledgecenter/#!/SSLKT6_7.5.0.5/com.ibm.mbs.doc/autoscript/c_automation_scripts.html ↩